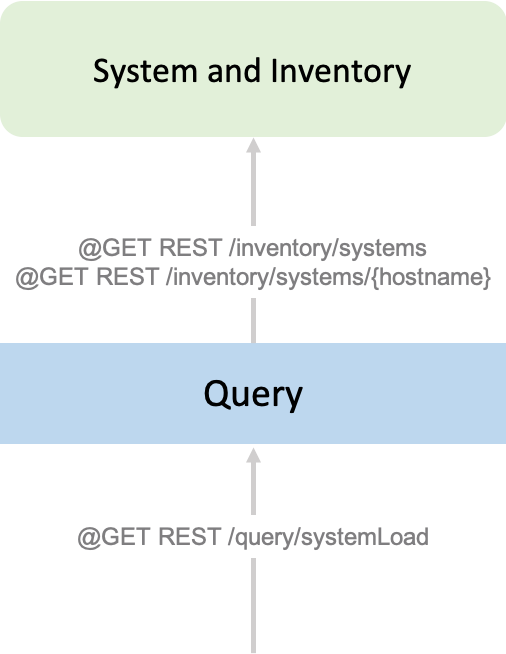
Contents
Tags
Consuming RESTful services asynchronously with template interfaces
Learn how to use MicroProfile Rest Client to invoke RESTful microservices asynchronously over HTTP.
What you’ll learn
You will learn how to build a MicroProfile Rest Client to access remote RESTful services using asynchronous method calls. You’ll update the template interface for a MicroProfile Rest Client to use the CompletionStage
return type. The template interface maps to the remote service that you want to call. A CompletionStage
interface allows you to work with the result of your remote service call asynchronously.
What is asynchronous programming?
Imagine asynchronous programming as a restaurant. After you’re seated, a waiter takes your order. Then, you must wait a few minutes for your food to be prepared. While your food is being prepared, your waiter may take more orders or serve other tables. After your food is ready, your waiter brings out the food to your table. However, in a synchronous model, the waiter must wait for your food to be prepared before serving any other customers. This method blocks other customers from placing orders or receiving their food.
You can perform lengthy operations, such as input/output (I/O), without blocking with asynchronous methods. The I/O operation can occur in the background and a callback notifies the caller to continue its computation when the original request is complete. As a result, the original thread frees up so it can handle other work rather than wait for the I/O to complete. Revisiting the restaurant analogy, food is prepared asynchronously in the kitchen and your waiter is freed up to attend to other tables.
In the context of REST clients, HTTP request calls can be time consuming. The network might be slow, or maybe the upstream service is overwhelmed and can’t respond quickly. These lengthy operations can block the execution of your thread when it’s in use and prevent other work from being completed.
The application in this guide consists of three microservices, system
, inventory
, and query
. Every 15 seconds the system
microservice calculates and publishes an event that contains its average system load. The inventory
microservice subscribes to that information so that it can keep an updated list of all the systems and their current system loads.
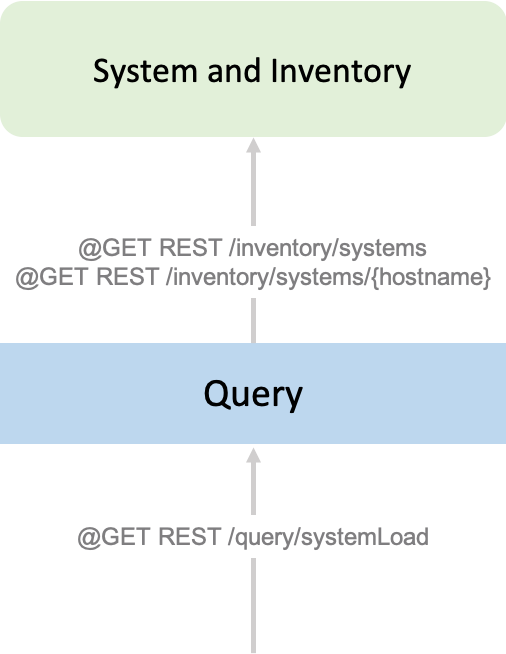
The microservice that you will modify is the query
service. It communicates with the inventory
service to determine which system has the highest system load and which system has the lowest system load.
The system
and inventory
microservices use MicroProfile Reactive Messaging to send and receive the system load events. If you want to learn more about reactive messaging, see the Creating Reactive Java Microservices guide.
Additional prerequisites
Before you begin, Docker needs to be installed. For installation instructions, refer to the official Docker documentation. You will build and run the microservices in Docker containers. An installation of Apache Kafka is provided in another Docker container.
Getting started
The fastest way to work through this guide is to clone the Git repository and use the projects that are provided inside:
git clone https://github.com/openliberty/guide-microprofile-rest-client-async.git
cd guide-microprofile-rest-client-async
The start
directory contains the starting project that you will build upon.
The finish
directory contains the finished project that you will build.
Before you begin, make sure you have all the necessary prerequisites.
Updating the template interface of a REST client to use asynchronous methods
Navigate to the start
directory to begin.
The query
service uses a MicroProfile Rest Client to access the inventory
service. You will update the methods in the template interface for this client to be asynchronous.
query/src/main/java/io/openliberty/guides/query/client/InventoryClient.java
The changes involve the getSystem
method. Change the return type to CompletionStage<Properties>
to make the method asynchronous. The method now has the return type of CompletionStage<Properties>
so you aren’t able to directly manipulate the Properties
inner type. As you will see in the next section, you’re able to indirectly use the Properties
by chaining callbacks.
Updating a REST resource to asynchronously handle HTTP requests
To reduce the processing time, you will update the /query/systemLoad
endpoint to asynchronously send the requests. Multiple client requests will be sent synchronously in a loop. The asynchronous calls do not block the program so the endpoint needs to ensure that all calls are completed and all returned data is processed before proceeding.
query/src/main/java/io/openliberty/guides/query/QueryResource.java
First, the systemLoad
endpoint first gets all the hostnames by calling getSystems()
. In the getSystem()
method, multiple requests are sent asynchronously to the inventory
service for each hostname. When the requests return, the thenAcceptAsync()
method processes the returned data with the CompletionStage<Properties>
interface.
The CompletionStage<Properties>
interface represents a unit of computation. After a computation is complete, it can either be finished or it can be chained with more CompletionStage<Properties>
interfaces using the thenAcceptAsync()
method. Exceptions are handled in a callback that is provided to the exceptionally()
method, which behaves like a catch block. When you return a CompletionStage<Properties>
type in the resource, it doesn’t necessarily mean that the computation completed and the response was built. JAX-RS responds to the caller after the computation completes.
In the systemLoad()
method a CountDownLatch
object is used to track asynchronous requests. The countDown()
method is called whenever a request is complete. When the CountDownLatch
is at zero, it indicates that all asynchronous requests are complete. By using the await()
method of the CountDownLatch
, the program waits for all the asynchronous requests to be complete. When all asynchronous requests are complete, the program resumes execution with all required data processed.
A Holder
class is used to wrap a variable called values
that has the volatile
keyword. The values
variable is instantiated as a ConcurrentHashMap
object. Together, the volatile
keyword and ConcurrentHashMap
type allow the Holder
class to store system information and safely access it asynchronously from multiple threads.
Building and running the application
You will build and run the system
, inventory
, and query
microservices in Docker containers. You can learn more about containerizing microservices with Docker in the Containerizing microservices guide.
Start your Docker environment. Dockerfiles are provided for you to use.
To build the application, run the Maven install
and package
goals from the command-line session in the start
directory:
WINDOWS
MAC
LINUX
mvnw.cmd -pl models install
mvnw.cmd package
Run the following commands to containerize the microservices:
docker build -t system:1.0-SNAPSHOT system/.
docker build -t inventory:1.0-SNAPSHOT inventory/.
docker build -t query:1.0-SNAPSHOT query/.
Next, use the provided startContainers
script to start the application in Docker containers. The script creates containers for Kafka and all of the microservices in the project, in addition to a network for the containers to communicate with each other. The script also creates three instances of the system
microservice.
WINDOWS
MAC
LINUX
.\scripts\startContainers.bat
The services take some time to become available. Visit the http://localhost:9085/health URL to confirm that the inventory
microservice is up and running.
You can access the application by making requests to the query/systemLoad
endpoint by going to the http://localhost:9080/query/systemLoad URL.
When the service is ready, you see an output similar to the following example which was formatted for readability.
{
"highest": {
"hostname" : "8841bd7d6fcd",
"systemLoad" : 6.96
},
"lowest": {
"hostname" : "37140ec44c9b",
"systemLoad" : 6.4
}
}
Switching to an asynchronous programming model freed up the thread that handles requests to the inventory
service. While requests process, the thread can handle other work or requests. In the /query/systemLoad
endpoint, multiple systems are read and compared at once.
When you are done checking out the application, run the following script to stop the application:
WINDOWS
MAC
LINUX
.\scripts\stopContainers.bat
Testing the query microservice
You will create an endpoint test to test the basic functionality of the query
microservice. If a test failure occurs, then you might have introduced a bug into the code.
query/src/test/java/it/io/openliberty/guides/query/QueryServiceIT.java
The testLoads()
test case verifies that the query
service can calculate the highest and lowest system loads.
Running the tests
Navigate to the start
directory, then verify that the tests pass by using the Maven verify
goal:
WINDOWS
MAC
LINUX
mvnw.cmd -pl query verify
When the tests succeed, you see output similar to the following example:
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Running it.io.openliberty.guides.query.QueryServiceIT
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 32.123 s - in it.io.openliberty.guides.query.QueryServiceIT
Results:
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0
Nice work! Where to next?
Nice work! You have just modified an application to make asynchronous HTTP requests using Open Liberty and MicroProfile Rest Client.
Consuming RESTful services asynchronously with template interfaces by Open Liberty is licensed under CC BY-ND 4.0
What did you think of this guide?




Thank you for your feedback!
What could make this guide better?
Raise an issue to share feedback
Create a pull request to contribute to this guide
Need help?
Ask a question on Stack Overflow