1[
2 {
3 "id": "1",
4 "name" : "foo",
5 "genres": "pop",
6 "albums" : [
7 {
8 "title" : "album_one",
9 "artist" : "foo",
10 "ntracks" : 12
11 },
12 {
13 "title" : "album_two",
14 "artist" : "foo",
15 "ntracks" : 15
16 }
17 ]
18 },
19 {
20 "id" : "2",
21 "name" : "bar",
22 "genres": "rock",
23 "albums" : [
24 {
25 "title" : "album_rock",
26 "artist" : "bar",
27 "ntracks" : 15
28 }
29 ]
30 },
31 {
32 "id": "3",
33 "name" : "dj",
34 "genres": "hip hop",
35 "albums" : [
36 {
37 "title" : "album_dj",
38 "artist" : "dj",
39 "ntracks" : 7
40 }
41 ]
42 },
43 {
44 "id": "4",
45 "name" : "flow",
46 "genres": "R&B",
47 "albums" : [
48 {
49 "title" : "album_long",
50 "artist" : "flow",
51 "ntracks" : 22
52 }
53 ]
54 },
55 {
56 "id": "5",
57 "name" : "cc",
58 "genres": "country",
59 "albums" : [
60 {
61 "title" : "album_short",
62 "artist" : "cc",
63 "ntracks" : 5
64 }
65 ]
66 },
67 {
68 "id": "6",
69 "name" : "axis",
70 "genres": "classical",
71 "albums" : [
72 {
73 "title" : "album_one",
74 "artist" : "axis",
75 "ntracks" : 9
76 },
77 {
78 "title" : "album_two",
79 "artist" : "axis",
80 "ntracks" : 8
81 },
82 {
83 "title" : "album_three",
84 "artist" : "axis",
85 "ntracks" : 11
86 }
87 ]
88 }
89]
Consuming a RESTful web service with ReactJS
Prerequisites:
Explore how to access a simple RESTful web service and consume its resources with ReactJS in Open Liberty.
What you’ll learn
You will learn how to access a REST service and deserialize the returned JSON that contains a list of artists and their albums by using an HTTP client with the ReactJS library. You will then present this data by using a ReactJS paginated table component.
ReactJS is a JavaScript library that is used to build user interfaces. Its main purpose is to incorporate a component-based approach to create reusable UI elements. With ReactJS, you can also interface with other libraries and frameworks. Note that the names ReactJS and React are used interchangeably.
The React application in this guide is provided and configured for you in the src/main/frontend
directory. The application uses the Create React App prebuilt configuration to set up the modern single-page React application. The create-react-app integrated toolchain is a comfortable environment for learning React and is the best way to start building a new single-page application with React.
artists.json
The REST service that provides the resources was written for you in advance in the back end of the application, and it responds with the artists.json
in the src/resources
directory. You will implement a ReactJS client as the front end of your application, which consumes this JSON file and displays its contents on a single-page webpage.
To learn more about REST services and how you can write them, see the Creating a RESTful web service guide.
Getting started
The fastest way to work through this guide is to clone the Git repository and use the projects that are provided inside:
git clone https://github.com/openliberty/guide-rest-client-reactjs.git
cd guide-rest-client-reactjs
The start
directory contains the starting project that you will build upon.
The finish
directory contains the finished project that you will build.
Before you begin, make sure you have all the necessary prerequisites.
Try what you’ll build
The finish
directory in the root of this guide contains the finished application. The React front end is already pre-built for you and the static files from the production build can be found in the src/main/webapp/static
directory.
To try out the application, navigate to the finish
directory and run the following Maven goal to build the application and deploy it to Open Liberty:
cd finish
mvn liberty:run
After you see the following message, your application Liberty instance is ready:
The defaultServer server is ready to run a smarter planet.
Next, point your browser to the http://localhost:9080 web application root to see the following output:
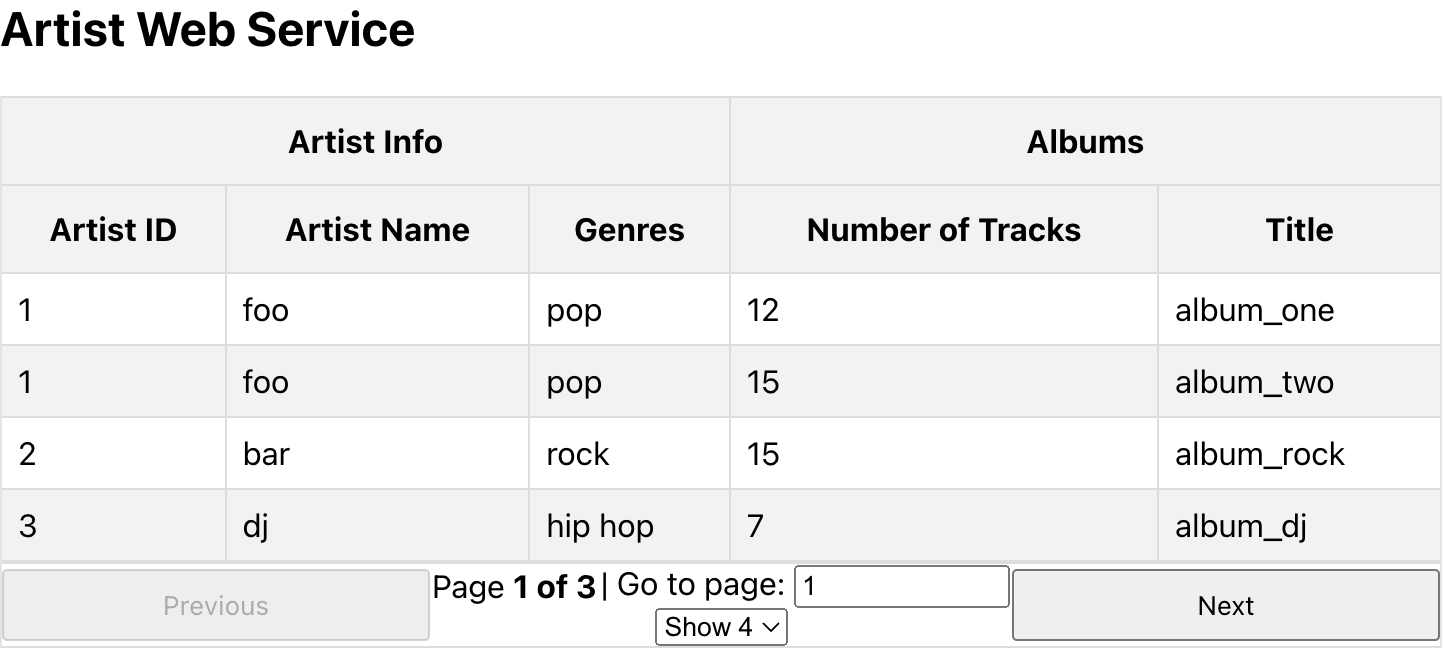
After you are finished checking out the application, stop the Liberty instance by pressing CTRL+C
in the command-line session where you ran Liberty. Alternatively, you can run the liberty:stop
goal from the finish
directory in another shell session:
mvn liberty:stop
Starting the service
Before you begin the implementation, start the provided REST service so that the artist JSON is available to you.
Navigate to the start
directory to begin.
When you run Open Liberty in dev mode, dev mode listens for file changes and automatically recompiles and deploys your updates whenever you save a new change. Run the following goal to start Open Liberty in dev mode:
mvn liberty:dev
After you see the following message, your Liberty instance is ready in dev mode:
************************************************************** * Liberty is running in dev mode.
Dev mode holds your command-line session to listen for file changes. Open another command-line session to continue, or open the project in your editor.
After you start the service, you can find your artist JSON at the http://localhost:9080/artists URL.
All the dependencies for the React front end can be found in src/main/frontend/src/package.json
, and they are installed before the front end is built by the frontend-maven-plugin
. Additionally, some provided CSS
stylesheets files are provided and can be found in the src/main/frontend/src/Styles
directory.
Project configuration
The front end of your application uses Node.js to build your React code. The Maven project is configured for you to install Node.js and produce the production files, which are copied to the web content of your application.
Node.js is a server-side JavaScript runtime that is used for developing networking applications. Its convenient package manager, npm, is used to run the React build scripts that are found in the package.json
file. To learn more about Node.js, see the official Node.js documentation.
The frontend-maven-plugin
is used to install
the dependencies that are listed in your package.json
file from the npm registry into a folder called node_modules
. The node_modules
folder can be found in your working
directory. Then, the configuration produces
the production files to the src/main/frontend/build
directory.
The maven-resources-plugin
copies the static
content from the build
directory to the web content
of the application.
pom.xml
Creating the default page
You need to create the entry point of your React application. create-react-app
uses the index.js
file as the main entry point of the application. This JavaScript file corresponds with the index.html
file, which is the entry point where your code runs in the browser.
Create theindex.js
file.src/main/frontend/src/index.js
index.js
1// tag::import-react[]
2import React from 'react';
3// end::import-react[]
4// tag::react-dom[]
5import ReactDOM from 'react-dom';
6// end::react-dom[]
7import './Styles/index.css';
8import App from './Components/App';
9
10// tag::dom-render[]
11ReactDOM.render(<App />, document.getElementById('root'));
12// end::dom-render[]
index.html
The React
library imports the react
package. A DOM, or Document Object Model, is a programming interface for HTML and XML documents. React offers a virtual DOM, which is essentially a copy of the browser DOM that resides in memory. The React virtual DOM improves the performance of your web application and plays a crucial role in the rendering process. The react-dom
package provides DOM-specific methods that can be used in your application to get outside of the React model, if necessary.
The render
method takes an HTML DOM element and tells the ReactDOM to render your React application inside of this DOM element. To learn more about the React virtual DOM, see the ReactDOM documentation.
Creating the React components
A React web application is a collection of components, and each component has a specific function. You will create the components that are used in the application to acquire and display data from the REST API.
The main component in your React application is the App
component. You need to create the App.js
file to act as a container for all other components.
Create theApp.js
file.src/main/frontend/src/Components/App.js
App.js
1import React from 'react';
2import {ArtistTable} from './ArtistTable';
3
4function App() {
5 return (
6 // tag::react-component[]
7 <ArtistTable/>
8 // end::react-component[]
9 );
10}
11
12export default App;
The App.js
file returns the ArtistTable
function to create a reusable element that encompasses your web application.
Next, create the ArtistTable
function that fetches data from your back end and renders it in a table.
Create theArtistTable.js
file.src/main/frontend/src/Components/ArtistTable.js
ArtistTable.js
The React
library imports the react
package for you to create the ArtistTable
function. This function must have the export
declaration because it is being exported to the App.js
module. The posts
object is initialized using a React Hook that lets you add a state to represent the state of the posts that appear on the paginated table.
To display the returned data, you will use pagination. Pagination is the process of separating content into discrete pages, and it can be used for handling data sets in React. In your application, you’ll render the columns in the paginated table. The columns
constant is used to define the table that is present on the webpage.
The useTable
hook creates a paginated table. The useTable
hook takes in the columns
, posts
, and setPosts
objects as parameters. It returns a paginated table that is assigned to the table
constant. The table
constant renders the paginated table on the webpage. The return
statement returns the paginated table. The useSortBy
hook sorts the paginated table by the column headers. The usePagination
hook creates the pagination buttons at the bottom of the table that are used to navigate through the paginated table.
Importing the HTTP client
Your application needs a way to communicate with and retrieve resources from RESTful web services to output the resources onto the paginated table. The Axios library will provide you with an HTTP client. This client is used to make HTTP requests to external resources. Axios is a promise-based HTTP client that can send asynchronous requests to REST endpoints. To learn more about the Axios library and its HTTP client, see the Axios documentation.
The GetArtistsInfo()
function uses the Axios API to fetch data from your back end. This function is called when the ArtistTable
is rendered to the page using the useEffect()
React lifecycle method.
Update theArtistTable.js
file.src/main/frontend/src/Components/ArtistTable.js
ArtistTable.js
1// tag::react-library[]
2import React, { useEffect, useMemo, useState } from 'react';
3// end::react-library[]
4// tag::axios-library[]
5import axios from 'axios';
6// end::axios-library[]
7// tag::react-table[]
8import { useTable, usePagination, useSortBy } from 'react-table';
9// end::react-table[]
10// tag::custom-style[]
11import '../Styles/table.css'
12// end::custom-styly[]
13
14// tag::ArtistTable[]
15export function ArtistTable() {
16 // end::ArtistTable[]
17
18 // tag::posts[]
19 const [posts, setPosts] = useState([]);
20 // end::posts[]
21
22 // tag::get-posts[]
23 const GetArtistsInfo = async () => {
24 // tag::axios[]
25 const response = await axios.get('http://localhost:9080/artists')
26 // end::axios[]
27 .then(response => {
28 // tag::response-data[]
29 const artists = response.data;
30 // end::response-data[]
31 // tag::for-artists[]
32 for (const artist of artists) {
33 // tag::spread-one[]
34 const { albums, ...rest } = artist;
35 // end::spread-one[]
36 for (const album of albums) {
37 //tag::setState[]
38 setPosts([...posts, { ...rest, ...album }]);
39 // end::setState[]
40 // tag::spread-two[]
41 posts.push({ ...rest, ...album });
42 // end::spread-two[]
43 }
44 };
45 // end::for-artists[]
46 }).catch(error => { console.log(error); });
47 };
48 // end::get-posts[]
49
50 // tag::useMemo[]
51 const data = useMemo(() => [...posts], [posts]);
52 // end::useMemo[]
53
54 // tag::table-info[]
55 const columns = useMemo(() => [{
56 Header: 'Artist Info',
57 columns: [
58 {
59 Header: 'Artist ID',
60 accessor: 'id'
61 },
62 {
63 Header: 'Artist Name',
64 accessor: 'name'
65 },
66 {
67 Header: 'Genres',
68 accessor: 'genres',
69 }
70 ]
71 },
72 {
73 Header: 'Albums',
74 columns: [
75 {
76 Header: 'Number of Tracks',
77 accessor: 'ntracks',
78 },
79 {
80 Header: 'Title',
81 accessor: 'title',
82 }
83 ]
84 }
85 ], []
86 );
87 // end::table-info[]
88
89 // tag::useTable[]
90 const tableInstance = useTable(
91 {
92 columns,
93 data,
94 initialState: { pageIndex: 0, pageSize: 4 }
95 },
96 // tag::useSortBy[]
97 useSortBy,
98 // end::useSortBy[]
99 // tag::usePagination[]
100 usePagination
101 // end::usePagination[]
102 )
103 // end::useTable[]
104
105 // tag::destructuring[]
106 const {
107 getTableProps,
108 getTableBodyProps,
109 headerGroups,
110 prepareRow,
111 page,
112 canPreviousPage,
113 canNextPage,
114 pageOptions,
115 pageCount,
116 gotoPage,
117 nextPage,
118 previousPage,
119 setPageSize,
120 state: { pageIndex, pageSize }
121 } = tableInstance;
122 // end::destructuring[]
123
124 // tag::useEffect[]
125 useEffect(() => {
126 GetArtistsInfo();
127 }, []);
128 // end::useEffect[]
129
130 // tag::return-table[]
131 return (
132 <>
133 <h2>Artist Web Service</h2>
134 {/* tag::table[] */}
135 <table {...getTableProps()}>
136 <thead>
137 {headerGroups.map(headerGroup => (
138 <tr {...headerGroup.getHeaderGroupProps()}>
139 {headerGroup.headers.map((column) => (
140 <th {...column.getHeaderProps(column.getSortByToggleProps())}>
141 {column.render('Header')}
142 <span>
143 {column.isSorted
144 ? column.isSortedDesc
145 ? ' 🔽'
146 : ' 🔼'
147 : ''}
148 </span>
149 </th>
150 ))}
151 </tr>
152 ))}
153 </thead>
154 <tbody {...getTableBodyProps()}>
155 {page.map((row, i) => {
156 prepareRow(row)
157 return (
158 <tr {...row.getRowProps()}>
159 {row.cells.map(cell => {
160 return <td {...cell.getCellProps()}>{cell.render('Cell')}</td>
161 })}
162 </tr>
163 )
164 })}
165 </tbody>
166 </table>
167 {/* end::table[] */}
168 <div className="pagination">
169 <button onClick={() => previousPage()} disabled={!canPreviousPage}>
170 {'Previous'}
171 </button>{' '}
172 <div className="page-info">
173 <span>
174 Page{' '}
175 <strong>
176 {pageIndex + 1} of {pageOptions.length}
177 </strong>{' '}
178 </span>
179 <span>
180 | Go to page:{' '}
181 <input
182 type="number"
183 defaultValue={pageIndex + 1}
184 onChange={e => {
185 const page = e.target.value ? Number(e.target.value) - 1 : 0
186 gotoPage(page)
187 }}
188 style={{ width: '100px' }}
189 />
190 </span>{' '}
191 <select
192 value={pageSize}
193 onChange={e => {
194 setPageSize(Number(e.target.value))
195 }}
196 >
197 {[4, 5, 6, 9].map(pageSize => (
198 <option key={pageSize} value={pageSize}>
199 Show {pageSize}
200 </option>
201 ))}
202 </select>
203 </div>
204 <button onClick={() => nextPage()} disabled={!canNextPage}>
205 {'Next'}
206 </button>{' '}
207 </div>
208 </>
209 );
210 // end::return-table[]
211}
Add the axios
library and the GetArtistsInfo()
function.
The axios
HTTP call is used to read the artist JSON that contains the data from the sample JSON file in the resources
directory. When a response is successful, the state of the system changes by assigning response.data
to posts
. The artists
and their albums
JSON data are manipulated to allow them to be accessed by the ReactTable
. The …rest
or …album
object spread syntax is designed for simplicity. To learn more about it, see Spread in object literals.
Building and packaging the front end
After you successfully build your components, you need to build the front end and package your application. The Maven process-resources
goal generates the Node.js resources, creates the front-end production build, and copies and processes the resources into the destination directory.
In a new command-line session, build the front end by running the following command in the start
directory:
mvn process-resources
The build may take a few minutes to complete. You can rebuild the front end at any time with the Maven process-resources
goal. Any local changes to your JavaScript and HTML are picked up when you build the front end.
Navigate to the http://localhost:9080 web application root to view the front end of your application.
Testing the React client
New projects that are created with create-react-app
comes with a test file called App.test.js
, which is included in the src/main/frontend/src
directory. The App.test.js
file is a simple JavaScript file that tests against the App.js
component. There are no explicit test cases that are written for this application. The create-react-app
configuration uses Jest as its test runner.
To learn more about Jest, go to their documentation on Testing React apps.
App.test.js
1import React from 'react';
2import { render } from '@testing-library/react';
3import App from './Components/App';
4
5test('renders artist web service title', () => {
6 const { getByText } = render(<App />);
7 const titleElement = getByText(/Artist Web Service/i);
8 expect(titleElement).toBeInTheDocument();
9});
Update thepom.xml
file.pom.xml
pom.xml
1<?xml version='1.0' encoding='utf-8'?>
2<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
3
4 <modelVersion>4.0.0</modelVersion>
5
6 <groupId>com.microprofile.demo</groupId>
7 <artifactId>guide-rest-client-reactjs</artifactId>
8 <version>1.0-SNAPSHOT</version>
9 <packaging>war</packaging>
10
11 <properties>
12 <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
13 <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
14 <maven.compiler.source>11</maven.compiler.source>
15 <maven.compiler.target>11</maven.compiler.target>
16 <!-- Liberty configuration -->
17 <liberty.var.http.port>9080</liberty.var.http.port>
18 <liberty.var.https.port>9443</liberty.var.https.port>
19 </properties>
20
21 <dependencies>
22 <!-- Provided dependencies -->
23 <dependency>
24 <groupId>jakarta.platform</groupId>
25 <artifactId>jakarta.jakartaee-api</artifactId>
26 <version>10.0.0</version>
27 <scope>provided</scope>
28 </dependency>
29 <dependency>
30 <groupId>org.eclipse.microprofile</groupId>
31 <artifactId>microprofile</artifactId>
32 <version>6.1</version>
33 <type>pom</type>
34 <scope>provided</scope>
35 </dependency>
36
37 <!-- For tests -->
38 <dependency>
39 <groupId>org.junit.jupiter</groupId>
40 <artifactId>junit-jupiter</artifactId>
41 <version>5.10.2</version>
42 <scope>test</scope>
43 </dependency>
44 </dependencies>
45
46 <build>
47 <finalName>${project.artifactId}</finalName>
48 <plugins>
49 <plugin>
50 <groupId>org.apache.maven.plugins</groupId>
51 <artifactId>maven-war-plugin</artifactId>
52 <version>3.4.0</version>
53 </plugin>
54 <!-- Enable liberty-maven plugin -->
55 <plugin>
56 <groupId>io.openliberty.tools</groupId>
57 <artifactId>liberty-maven-plugin</artifactId>
58 <version>3.10.2</version>
59 </plugin>
60 <!-- Frontend resources -->
61 <!-- tag::frontend-plugin[] -->
62 <plugin>
63 <groupId>com.github.eirslett</groupId>
64 <artifactId>frontend-maven-plugin</artifactId>
65 <version>1.15.0</version>
66 <configuration>
67 <!-- tag::working-dir[] -->
68 <workingDirectory>src/main/frontend</workingDirectory>
69 <!-- end::working-dir[] -->
70 </configuration>
71 <executions>
72 <execution>
73 <id>install node and npm</id>
74 <goals>
75 <goal>install-node-and-npm</goal>
76 </goals>
77 <configuration>
78 <nodeVersion>v21.6.2</nodeVersion>
79 <npmVersion>10.2.4</npmVersion>
80 </configuration>
81 </execution>
82 <!-- tag::node-resource-install[] -->
83 <execution>
84 <id>npm install</id>
85 <goals>
86 <goal>npm</goal>
87 </goals>
88 <configuration>
89 <arguments>install</arguments>
90 </configuration>
91 </execution>
92 <!-- end::node-resource-install[] -->
93 <!-- tag::node-resource-build[] -->
94 <execution>
95 <id>npm run build</id>
96 <goals>
97 <goal>npm</goal>
98 </goals>
99 <configuration>
100 <arguments>run build</arguments>
101 </configuration>
102 </execution>
103 <!-- end::node-resource-build[] -->
104 <!-- tag::node-tests[] -->
105 <execution>
106 <id>run tests</id>
107 <goals>
108 <goal>npm</goal>
109 </goals>
110 <configuration>
111 <arguments>test a</arguments>
112 <environmentVariables>
113 <CI>true</CI>
114 </environmentVariables>
115 </configuration>
116 </execution>
117 <!-- end::node-tests[] -->
118 </executions>
119 </plugin>
120 <!-- end::frontend-plugin[] -->
121 <!-- Copy frontend static files to target directory -->
122 <!-- tag::copy-plugin[] -->
123 <plugin>
124 <groupId>org.apache.maven.plugins</groupId>
125 <artifactId>maven-resources-plugin</artifactId>
126 <version>3.3.1</version>
127 <executions>
128 <execution>
129 <id>Copy frontend build to target</id>
130 <phase>process-resources</phase>
131 <goals>
132 <goal>copy-resources</goal>
133 </goals>
134 <configuration>
135 <outputDirectory>
136 <!-- tag::output-directory[] -->
137 ${basedir}/src/main/webapp
138 <!-- end::output-directory[] -->
139 </outputDirectory>
140 <resources>
141 <resource>
142 <directory>
143 <!-- tag::directory[] -->
144 ${basedir}/src/main/frontend/build
145 <!-- end::directory[] -->
146 </directory>
147 <filtering>true</filtering>
148 </resource>
149 </resources>
150 </configuration>
151 </execution>
152 </executions>
153 </plugin>
154 <!-- end::copy-plugin[] -->
155 </plugins>
156 </build>
157</project>
To run the default test, you can add the testing
configuration to the frontend-maven-plugin
. Rerun the Maven process-resources
goal to rebuild the front end and run the tests.
Although the React application in this guide is simple, when you build more complex React applications, testing becomes a crucial part of your development lifecycle. If you need to write application-oriented test cases, follow the official React testing documentation.
When you are done checking the application root, exit dev mode by pressing CTRL+C
in the shell session where you ran the Liberty.
Great work! You’re done!
Nice work! You just accessed a simple RESTful web service and consumed its resources by using ReactJS in Open Liberty.
Guide Attribution
Consuming a RESTful web service with ReactJS by Open Liberty is licensed under CC BY-ND 4.0
Prerequisites:
Nice work! Where to next?
What did you think of this guide?




Thank you for your feedback!
What could make this guide better?
Raise an issue to share feedback
Create a pull request to contribute to this guide
Need help?
Ask a question on Stack Overflow