git clone https://github.com/openliberty/guide-contract-testing.git
cd guide-contract-testing
Contents
Tags
Testing microservices with consumer-driven contracts
Learn how to test Java microservices with consumer-driven contracts in Open Liberty.
What you’ll learn
With a microservices-based architecture, you need robust testing to ensure that microservices that depend on one another are able to communicate effectively. Typically, to prevent multiple points of failure at different integration points, a combination of unit, integration, and end-to-end tests are used. While unit tests are fast, they are less trustworthy because they run in isolation and usually rely on mock data.
Integration tests address this issue by testing against real running services. However, they tend to be slow as the tests depend on other microservices and are less reliable because they are prone to external changes.
Usually, end-to-end tests are more trustworthy because they verify functionality from the perspective of a user. However, a graphical user interface (GUI) component is often required to perform end-to-end tests, and GUI components rely on third-party software, such as Selenium, which requires heavy computation time and resources.
What is contract testing?
Contract testing bridges the gaps among the shortcomings of these different testing methodologies. Contract testing is a technique for testing an integration point by isolating each microservice and checking whether the HTTP requests and responses that the microservice transmits conform to a shared understanding that is documented in a contract. This way, contract testing ensures that microservices can communicate with each other.
Pact is an open source contract testing tool for testing HTTP requests, responses, and message integrations by using contract tests.
The Pact Broker is an application for sharing Pact contracts and verification results. The Pact Broker is also an important piece for integrating Pact into continuous integration and continuous delivery (CI/CD) pipelines.
The two microservices you will interact with are called system
and inventory
. The system
microservice returns the JVM system properties of its host. The inventory
microservice retrieves specific properties from the system
microservice.
You will learn how to use the Pact framework to write contract tests for the inventory
microservice that will then be verified by the system
microservice.
Additional prerequisites
Before you begin, you need to install Docker if it is not already installed. For installation instructions, refer to the official Docker documentation. You will deploy the Pact Broker in a Docker container.
Start your Docker daemon before you proceed.
Getting started
The fastest way to work through this guide is to clone the Git repository and use the projects that are provided inside:
The start
directory contains the starting project that you will build upon.
The finish
directory contains the finished project that you will build.
Before you begin, make sure you have all the necessary prerequisites.
Starting the Pact Broker
Run the following command to start the Pact Broker:
docker compose -f "pact-broker/docker-compose.yml" up -d --build
When the Pact Broker is running, you’ll see the following output:
...
Container pact-broker-postgres-1 Started
Container pact-broker-pact-broker-1 Started
Go to the http://localhost:9292/ URL to confirm that you can access the user interface (UI) of the Pact Broker.
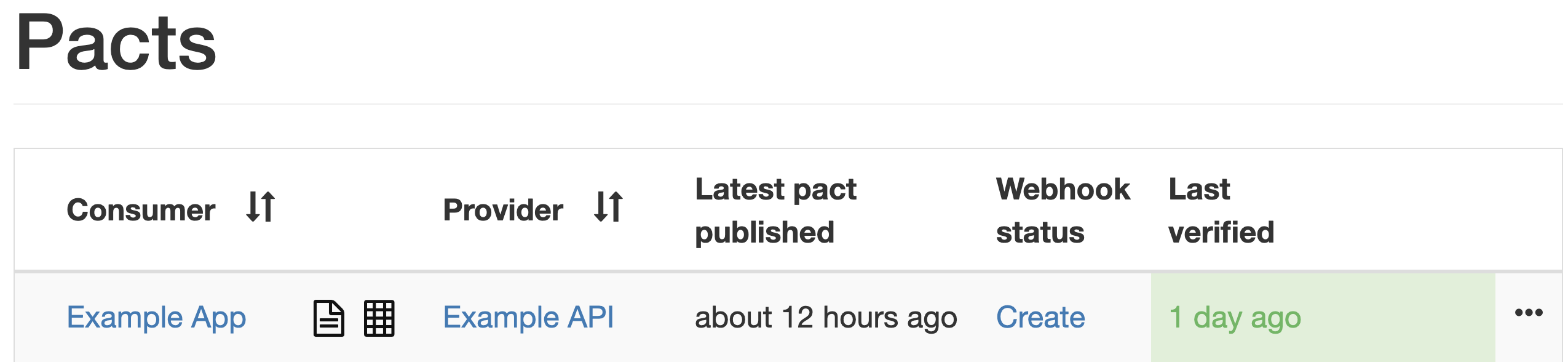
You can refer to the official Pact Broker documentation for more information about the components of the Docker Compose file.
Implementing pact testing in the inventory service
Navigate to the start
directory to begin.
When you run Open Liberty in dev mode, dev mode listens for file changes and automatically recompiles and deploys your updates whenever you save a new change. Run the following goal to start Open Liberty in dev mode:
WINDOWS
MAC
LINUX
mvnw.cmd -f inventory\pom.xml liberty:dev
After you see the following message, your Liberty instance is ready in dev mode:
************************************************************** * Liberty is running in dev mode.
Dev mode holds your command-line session to listen for file changes. Open another command-line session to continue, or open the project in your editor.
inventory/src/test/java/io/openliberty/guides/inventory/InventoryPactIT.java
The InventoryPactIT
class contains a PactProviderRule
mock provider that mimics the HTTP responses from the system
microservice. The @Pact
annotation takes the name of the microservice as a parameter, which makes it easier to differentiate microservices from each other when you have multiple applications.
The createPactServer()
method defines the minimal expected responses for a specific endpoint, which is known as an interaction. For each interaction, the expected request and the response are registered with the mock service by using the @PactVerification
annotation.
The test sends a real request with the getUrl()
method of the mock provider. The mock provider compares the actual request with the expected request and confirms whether the comparison is successful. Finally, the assertEquals()
method confirms that the response is correct.
inventory/pom.xml
The Pact framework provides a maven
plugin that can be added to the build section of the pom.xml
file. The serviceProvider
element defines the endpoint URL for the system
microservice and the pactFileDirectory
directory where you want to store the pact file. The pact-jvm-consumer-junit
dependency provides the base test class that you can use with JUnit to build unit tests.
After you create the InventoryPactIT.java
class and replace the pom.xml
file, Open Liberty automatically reloads its configuration.
The contract between the inventory
and system
microservices is known as a pact. Each pact is a collection of interactions. In this guide, those interactions are defined in the InventoryPactIT
class.
Press the enter/return
key to run the tests and generate the pact file from the command-line session where you started the inventory
microservice.
When completed, you’ll see a similar output to the following example:
[INFO] -------------------------------------------------------
[INFO] T E S T S
[INFO] -------------------------------------------------------
[INFO] Running io.openliberty.guides.inventory.InventoryPactIT
[INFO] Tests run: 4, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 1.631 s - in io.openliberty.guides.inventory.InventoryPactIT
[INFO]
[INFO] Results:
[INFO]
[INFO] Tests run: 4, Failures: 0, Errors: 0, Skipped: 0
When you integrate the Pact framework in a CI/CD build pipeline, you can use the Maven failsafe:integration-test
goal to generate the pact file. The Maven failsafe
plug-in provides a lifecycle phase for running integration tests that run after unit tests. By default, it looks for classes that are suffixed with IT
, which stands for Integration Test. You can refer to the Maven failsafe plug-in documentation for more information.
The generated pact file is named Inventory-System.json
and is located in the inventory/target/pacts
directory. The pact file contains the defined interactions in JSON format:
{ ... "interactions": [ { "description": "a request for server name", "request": { "method": "GET", "path": "/system/properties/key/wlp.server.name" }, "response": { "status": 200, "headers": { "Content-Type": "application/json" }, "body": [ { "wlp.server.name": "defaultServer" } ] }, "providerStates": [ { "name": "wlp.server.name is defaultServer" } ] } ... ] }
Now, navigate to the start
directory again.
Publish the generated pact file to the Pact Broker by running the following command:
WINDOWS
MAC
LINUX
mvnw.cmd -f inventory\pom.xml pact:publish
After the file is published, you’ll see a similar output to the following example:
--- maven:4.1.21:publish (default-cli) @ inventory ---
Publishing 'Inventory-System.json' with tags 'open-liberty-pact' ... OK
Verifying the pact in the Pact Broker
Refresh the Pact Broker webpage at the http://localhost:9292/ URL to verify that a new entry exists. The last verified column doesn’t show a timestamp because the system
microservice hasn’t verified the pact yet.
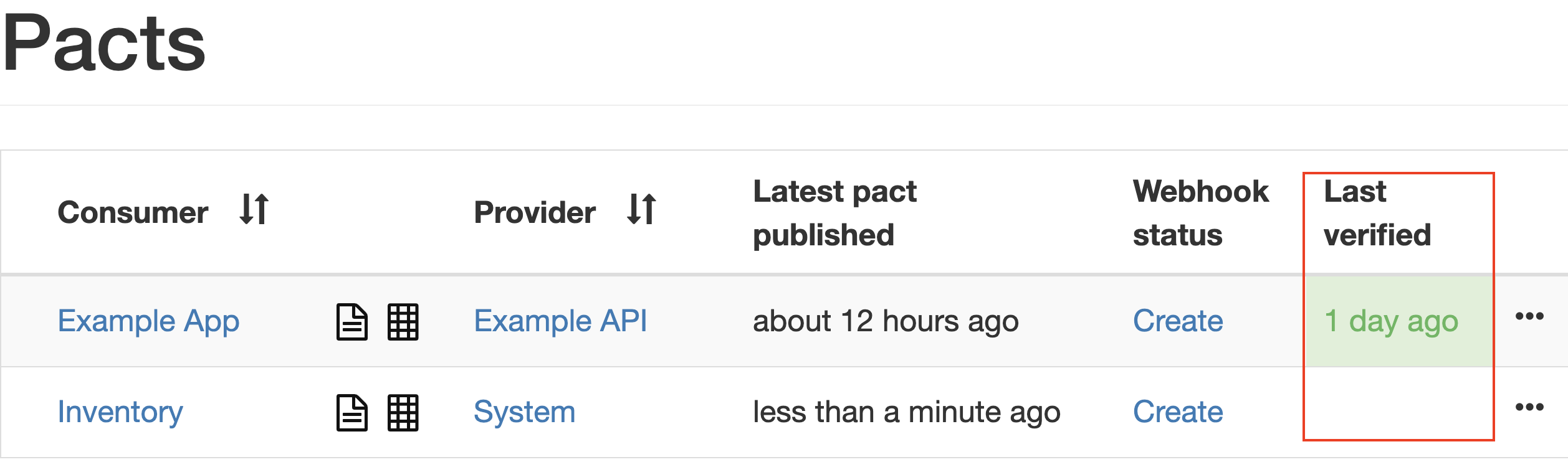
You can see detailed insights about each interaction by going to the http://localhost:9292/pacts/provider/System/consumer/Inventory/latest URL, as shown in the following image:
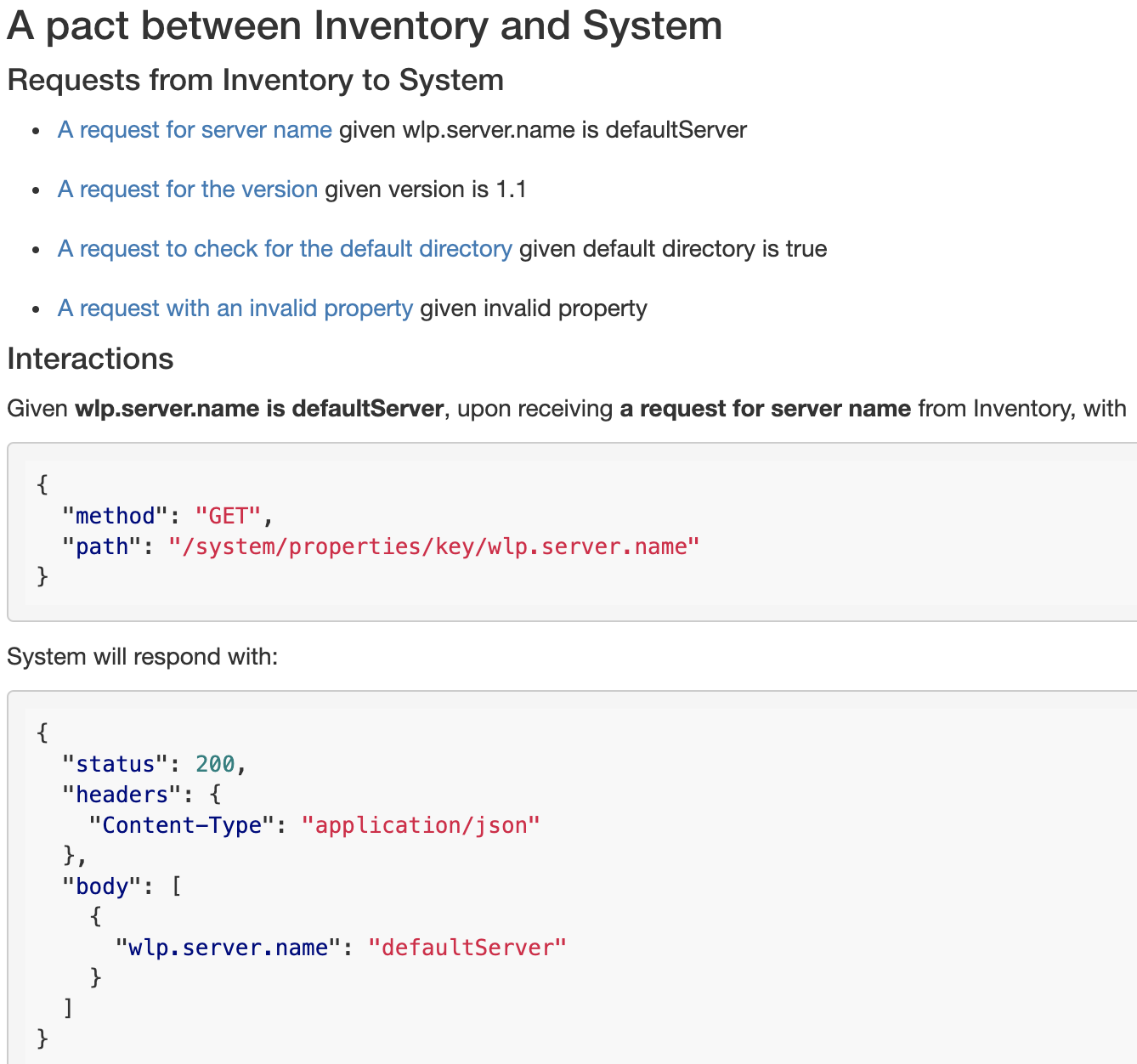
Implementing pact testing in the system service
Open another command-line session and navigate to the start
directory.
Start Open Liberty in dev mode for the system
microservice:
WINDOWS
MAC
LINUX
mvnw.cmd -f system\pom.xml liberty:dev
After you see the following message, your Liberty instance is ready in dev mode:
************************************************************** * Liberty is running in dev mode.
Open another command-line session and navigate to the start
directory to continue.
system/src/test/java/it/io/openliberty/guides/system/SystemBrokerIT.java
The connection information for the Pact Broker is provided with the @PactBroker
annotation. The dependency also provides a JUnit5 Invocation Context Provider with the pactVerificationTestTemplate()
method to generate a test for each of the interactions.
The pact.verifier.publishResults
property is set to true
so that the results are sent to the Pact Broker after the tests are completed.
The test target is defined in the PactVerificationContext
context to point to the running endpoint of the system
microservice.
The @State
annotation must match the given()
parameter that was provided in the inventory
test class so that Pact can identify which test case to run against which endpoint.
system/pom.xml
The system
microservice uses the junit5
pact provider dependency to connect to the Pact Broker and verify the pact file. Ideally, in a CI/CD build pipeline, the pact.provider.version
element is dynamically set to the build number so that you can identify where a breaking change is introduced.
After you create the SystemBrokerIT.java
class and replace the pom.xml
file, Open Liberty automatically reloads its configuration.
Verifying the contract
In the command-line session where you started the system
microservice, press the enter/return
key to run the tests to verify the pact file. When you integrate the Pact framework into a CI/CD build pipeline, you can use the Maven failsafe:integration-test
goal to verify the pact file from the Pact Broker.
The tests fail with the following errors:
[ERROR] Failures:
[ERROR] SystemBrokerIT.pactVerificationTestTemplate:28 Pact between Inventory (1.0-SNAPSHOT) and System - Upon a request for the version
Failures:
1) Verifying a pact between Inventory and System - a request for the version has a matching body
1.1) body: $.system.properties.version Expected "1.x" (String) to be a decimal number
[INFO]
[ERROR] Tests run: 4, Failures: 1, Errors: 0, Skipped: 0
The test from the system
microservice fails because the inventory
microservice was expecting a decimal, 1.1
, for the value of the system.properties.version
property, but it received a string, "1.1"
.
Correct the value of the system.properties.version
property to a decimal.
system/src/main/java/io/openliberty/guides/system/SystemResource.java
Press the enter/return
key to rerun the tests from the command-line session where you started the system
microservice.
If the tests are successful, you’ll see a similar output to the following example:
...
Verifying a pact between pact between Inventory (1.0-SNAPSHOT) and System
Notices:
1) The pact at http://localhost:9292/pacts/provider/System/consumer/Inventory/pact-version/XXX is being verified because it matches the following configured selection criterion: latest pact for a consumer version tagged 'open-liberty-pact'
[from Pact Broker http://localhost:9292/pacts/provider/System/consumer/Inventory/pact-version/XXX]
Given version is 1.1
a request for the version
returns a response which
has status code 200 (OK)
has a matching body (OK)
[main] INFO au.com.dius.pact.provider.DefaultVerificationReporter - Published verification result of 'au.com.dius.pact.core.pactbroker.TestResult$Ok@4d84dfe7' for consumer 'Consumer(name=Inventory)'
[INFO] Tests run: 4, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 1.835 s - in it.io.openliberty.guides.system.SystemBrokerIT
...
After the tests are complete, refresh the Pact Broker webpage at the http://localhost:9292/ URL to confirm that the last verified column now shows a timestamp:
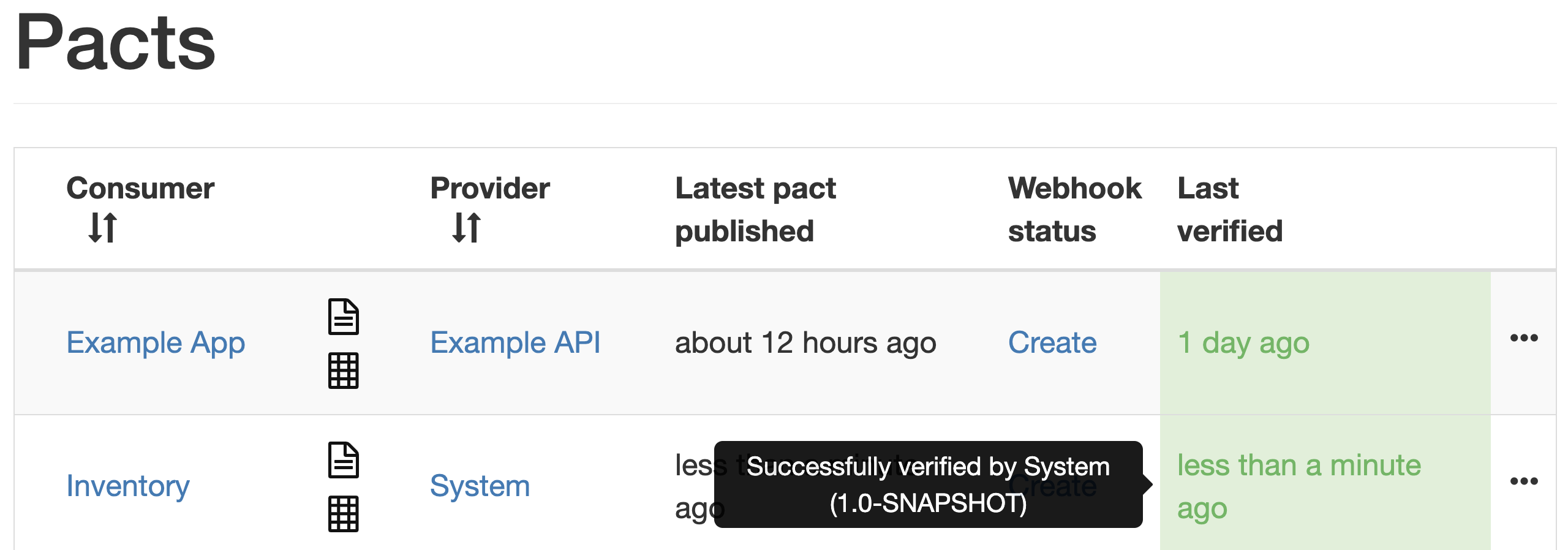
The pact file that’s created by the inventory
microservice was successfully verified by the system
microservice through the Pact Broker. This ensures that responses from the system
microservice meet the expectations of the inventory
microservice.
Tearing down the environment
When you are done checking out the service, exit dev mode by pressing CTRL+C
in the command-line sessions where you ran the Liberty instances for the system
and inventory
microservices.
Navigate back to the /guide-contract-testing
directory and run the following commands to remove the Pact Broker:
docker compose -f "pact-broker/docker-compose.yml" down
docker rmi postgres:17.2
docker rmi pactfoundation/pact-broker:latest
docker volume rm pact-broker_postgres-volume
Nice work! Where to next?
Nice work! You implemented contract testing in Java microservices by using Pact and verified the contract with the Pact Broker.
Testing microservices with consumer-driven contracts by Open Liberty is licensed under CC BY-ND 4.0
What did you think of this guide?




Thank you for your feedback!
What could make this guide better?
Raise an issue to share feedback
Create a pull request to contribute to this guide
Need help?
Ask a question on Stack Overflow